· 13 min read Posted by Kevin Galligan
Kotlin Native Stranger Threads Ep 1
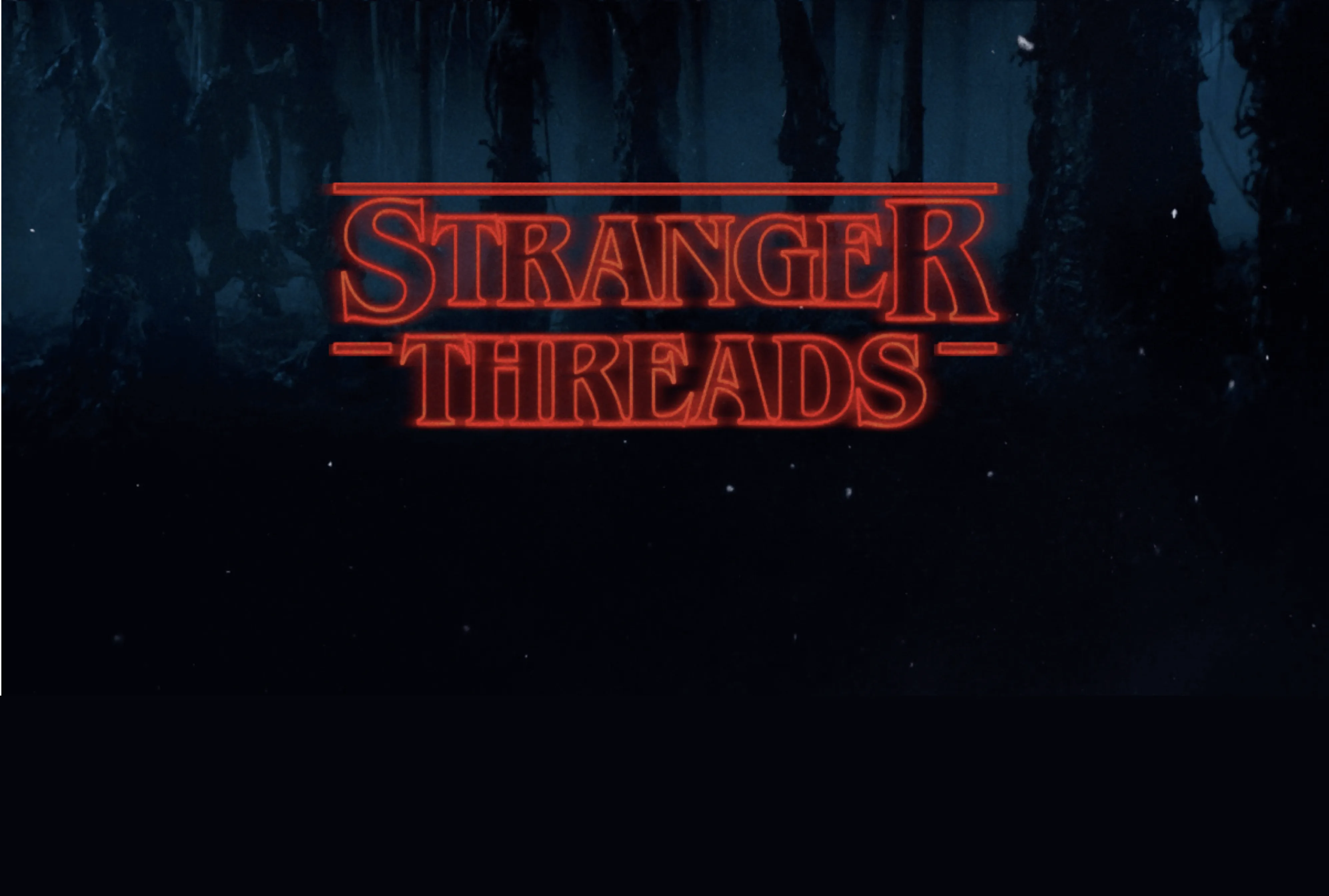
Episode 1 — Worker
My original post about Kotlin Native (KN) concurrency was written a while ago, with a much earlier version of Native and Multiplatform. Now that Kotlin Multiplatform is ready for production development, it’s time to revisit how Native concurrency works and how to use it in your application development.
Concurrency and state in KN is significantly different compared to what you’re likely used to. Languages like Java, Swift, Objective-C, and C++ give the developer tools to ensure proper concurrent state access, but using them properly is up to the developer. Writing concurrent code in these languages can be difficult and error prone. KN, by contrast, introduces constraints that allow the runtime to verify that concurrent access is safe, while also providing for reasonable flexibility. It is trying to find a balance between safety and access. What that means is changing, and even within Jetbrains there appear to be conflicting visions. What is clear, however, is that Jetbrains is committed to Saner Concurrency, and to building a platform for the future.
In this series we’ll cover the rules and structures of KN’s concurrency and state model, and how they apply in the context of application development.
Just FYI, if you see emoji in the doc, that’s generally a footnote with unnecessary info 😛.
Episode 1 — Workers ⏮
Kotlin Native (KN) concurrency is kind of a big topic. For developers familiar with Java and Swift/ObjC concurrency, there are several new concepts to learn, which presents a problem out of the gate. Where to start?
In general, I like to be able to play with the code right away, so we’ll start with a core KN concurrency mechanism: Worker. We’ll encounter some concepts before we’ve had a chance to explain them, but we’ll sort that out later on in the series.
The code samples in this post can be found here. You’ll need to have a MacOS machine to run them. Adding other platforms should be pretty simple, if anybody wants to give it a shot.
Most of the examples are implemented as unit tests 🔍. You can run them by typing:
./gradlew build
Worker
KN supports concurrency out of the box using a structure called “Worker”. A Worker is a job queue on which you can schedule jobs to run on a different thread.
The Worker related tests can be found here.
Creating a worker is relatively straightforward.
import kotlin.native.concurrent.Worker
class TestWorker {
val worker = Worker.start()
}
Each Worker instance gets a thread 📄. You can schedule jobs to be run on the worker’s thread.
worker.execute(TransferMode.SAFE, {"Hello"}) {
//Do something on Worker thread
}
There are a few things to take note of in that call. Here’s the function definition for execute:
fun <T1, T2> execute(
mode: TransferMode,
producer: () -> T1,
job: (T1) -> T2): Future<T2>
We’ll discuss TransferMode in part 2. In summary, there are two options: SAFE and UNSAFE. Just assume it’s always TransferMode.SAFE.
The producer parameter is a lambda that returns the input to the background job (generic type T1). That’s how you pass data to your background task.
It’s critically important to understand that whatever gets returned from the producer lambda is intended to be passed to another thread, and as a result, must follow KN state and concurrency rules. That means it either needs to be frozen, or needs to be fully detachable. In theory, being detachable is simple, but in practice it can be tricky. We’ll talk about that in a bit.
The job parameter is the work you intend to do on the background thread. It will take the result of the producer (T1) as a parameter and return a result (T2) that will be available from the Future.
Well discuss this more later on, but it’s a super important topic and can bear some repetition. It is very easy to accidentally capture outside state in the job lambda. This is not allowed and the compiler will complain. You’ll need to be extra careful to avoid doing that.
Execute’s return is ‘Future<T2>’. Your calling thread can block and wait for this value, but in an interactive application we’ll need a way back to the calling context that doesn’t interrupt the ui.
producer
The producer’s job is very simple. Isolate a parameter value to hand off to the background job. You’ll see the producer lambda both here and when we need to detach an object from the object graph. It’s a little confusing at first, but understanding what’s happening with the producer will help clear up KN’s broader concurrency concepts.
Take note of the fact that the producer is a lambda and not just a value. It doesn’t look like this.
worker.execute(TransferMode.SAFE, "Hello"){
//Do something
}
That is (presumably) to make isolating and detaching the object reference easier.
The producer is run in whatever thread you’re calling it from.The result of that lambda is then checked to make sure it can be safely given to the worker’s thread. However, to be clear, all of that activity happens in your current thread. We only engage the worker’s thread when we get to the background job.
Haven’t left the calling thread yet
How do we determine that some state can be safely given to another thread? We have to respect KN’s two basic rules:
-
- Live state belongs to one thread
-
Frozen state can be shared
Part two is all about the two rules, but in summary:
-
- Live state is the state you’re used to writing
-
Frozen is, basically, super-immutable. You create frozen state by calling ‘freeze’ on it
Note: We’ll start using data classes rather than String. Strings, as well as other basic value types, are frozen automatically by the runtime.
Here’s a basic example:
data class JobArg(val a: String)
@Test
fun simpleProducer() {
worker.execute(TransferMode.SAFE, { JobArg("Hi") }) {
println(it)
}
}
We create an instance of JobArg inside the producer. There are no external references (nobody has a reference to that instance of JobArg), so the runtime can safely detach and pass the state to the job lambda to be run in another thread.
This, by contrast, fails.
@Test
fun frameReferenceFails() {
val valArg = JobArg("Hi")
assertFails {
worker.execute(TransferMode.SAFE, { valArg }) {
println(it)
}
}
}
When we call execute, valArg is being referenced locally, so the attempt to detach will fail.
This looks like a way to hide the reference, but also fails:
class ArgHolder(var arg:JobArg?){
fun getAndClear():JobArg{
val temp = arg!!
arg = null
return temp
}
}
@Test
fun stillVisible() {
val holder = ArgHolder(JobArg("Hi"))
assertFails {
worker.execute(TransferMode.SAFE, { holder.getAndClear() }) {
println(it)
}
}
}
Why? Well, this gets a bit into the weeds of how KN’s memory model works. Native doesn’t use a garbage collector 🚮. It uses reference counting. Each allocated object has a count of how many other entities have a reference to it. When that count goes to zero, that memory is freed.
iOS developers will have an easier time with this concept, as this is how Swift and ObjC work 🍎.
References to objects obviously include hard field references, but also include local frame references. That’s what’s wrong with the block above. The JobArg appears in the local frame context, however briefly, which still has a reference to it when the producer attempts to detach it.
Outside context has a local reference
This, however, will work:
fun makeInstance() = ArgHolder(JobArg("Hi"))
@Test
fun canDetach() {
val holder = makeInstance()
worker.execute(TransferMode.SAFE, { holder.getAndClear() }) {
println(it)
}
}
The local ref is cleared in ‘makeInstance’. So again, if you’re wondering why the producer is a lambda, it’s to make avoiding local references easier. Look at simpleProducer again:
@Test
fun simpleProducer() {
worker.execute(TransferMode.SAFE, { JobArg("Hi") }) {
println(it)
}
}
Much simpler.
Confused?
Passing live data is difficult syntactically. In fact, we don’t have multithreaded coroutines yet because JB still needs to reconcile the two systems 😟. I gave you some pretty weird examples out of the gate on purpose. KN makes passing mutable state between threads difficult, and in general that’s a good thing, because it’s risky. When I need to pass something into a worker I’ll almost always freeze it.
@Test
fun frozenFtw() {
val valArg = JobArg("Hi").freeze()
worker.execute(TransferMode.SAFE, { valArg }) {
println(it)
}
}
Because frozen data can be shared between threads, the producer can return valArg. This is obviously a simple example, but as you get into Native development, you’ll generally find freezing data to be simpler, and in general, data that you’re passing around should be immutable anyway.
I should mention that you can bypass all of this and simply pass data unsafe with TransferMode.UNSAFE, and it’ll probably work most of the time. Don’t do it, though. It’s called UNSAFE for a reason, so if you can’t clearly explain why you would use it, you never should. We’ll discuss this in detail in part 2.
We spent a lot of time on the producer, but again, the producer introduces a lot of core, and potentially confusing topics. If you can fully grasp what’s going on with that you’ll have covered a lot of ground.
Background Job
What happens with the background lambda, compared to what was happening with the producer, is much simpler. The lambda takes a single parameter, which is the result of the producer (which, btw, can be empty). If the background job returns a value, it’ll be available from the Future.
@Test
fun backgroundStuff() {
val future = worker.execute(TransferMode.SAFE, { 1000000 }) {
var count = 0
for(i in 1..it){
//Do some long stuff
count++
}
count
}
assertEquals(1000000, future.result)
}
Here we’re going to loop and count. We pass the number of loops in the producer.
Just FYI, be careful with threads and unit tests 🍌. The ‘future.result’ forces the thread to wait for the background lambda to finish.
Until now, everything happened in the original calling context. The background job finally gets us into the second thread.
Since job is in a different thread, you can’t reference just any state. Only the lambda param of type T1, originally from our friend producer, and global state known to be frozen or thread local. In other words, only state that the KN runtime can verify is safe to access.
As mentioned previously, it’s pretty easy to capture other state in the lambda of your background task. The compiler attempts to prevent this, but only when you’re calling the worker method directly. We’ll dive deeper into that when we talk about actually implementing concurrency in your applications.
In simple examples, capturing extra state won’t be much of a problem. Where this quickly becomes problematic is capturing state when you call background tasks from your application objects. I found this difficult at first, but you get used to it. Frameworks help, and especially when multithreaded coroutines become available, running tasks in the background will be simpler 😴.
Future
The ‘execute’ method returns a Future instance, which can be used to check the status of the background process, as well as get the value returned. The value can be Unit, which means you’ll simply verify that the process completed.
If it’s OK to block the calling thread, the simplest way to get your result is to call the result property on the Future instance. That’s what we’re doing in the test examples.
Alternatively you can poll status on the Future, or set up a result Worker to call back to. However, if you’re intending to use Worker in the context of a mobile application, going “back to the main thread” is somewhat more complex. We’ll discuss that later.
Lifecycle
We don’t worry about it too much in the context of our test samples, but you should shut down Workers when you’re done with them. This is only necessary if you’re going to keep the process running but abandon the Worker. It your Worker instances are meant to live along with your process, you can leave them hanging around (they get shut down with the process).
@Test
fun requestTermination(){
val w = Worker.start()
w.requestTermination().result
}
requestTermination returns a Future. If you need to wait for termination, check the result.
You Probably Won’t Use Worker
In the same way you probably don’t create a Thread instance or an ExecutorService very often in Java, libraries will probably keep you away from creating Worker instances directly. Unless KN state rules radically change, however, you won’t get away from those. You will, however, be seeing Worker a lot for the next few posts at least.
Up Next
Worker introduces us to the basics of running concurrent code on Native. Part 2 will go deeper into the why of KN state rules, freezing, detaching, and some more detail about what’s happening under the hood.
😛 But super interesting info!!!
⏮ OK. It’s not exactly Episode 1. The earlier post, from about 8 months ago, was supposed to be the start of the series, but things were changing really fast and I got more involved in library development. Yada yada, we’ll call that the pilot and this is the start ot the series.
🔍 The test code is configured with a common source set and a native source set. To get native code tests to run on the command line, the simplest way to do that is to build a macos target. The build process automatically builds and runs a command line executable. JVM is currently disabled because we’re not talking about the JVM 🙂
📄 The docs are pretty clear that you shouldn’t rely on that in the future as it may change, but for the foreseeable future, 1 Worker gets one thread.
🚮 That’s mostly true. There is a garbage collector in the runtime, but I’m pretty sure that’s there to deal with reference cycles. Memory is primarily managed by reference counting.
🍎 There are some important differences to note. KN can deal with reference cycles, so “weak” references aren’t a concern. Also, to be clear, KN objects are ref counted, and it’s conceptually similar to ARC, but it’s a separate system. While running on iOS, KN doesn’t use ARC for it’s ref counts.
😟 A fair number of people have expressed their hope that JB abandons the “Saner Concurrency” effort. The comment in that coroutines issue implies they might, or at least relax the rules somewhat. While I understand this stuff can be confusing, the ultimate goal is to produce a better platform. I would very much like some improved debug info from immutability related exceptions, and some improved library support, but once you get your head around this stuff it’s not that bad.
🍌 Calling for the future result forces the main thread to wait. That’s why this test works correctly. This can all get very tricky when trying to interact with the main thread, etc. There are frameworks and examples in more mature ecosystems to help out, but KN and multiplatform are in early days. Just an FYI.
😴 I’ve been asked if there’s any reason to learn this crazy threading stuff if the coroutines API will largely hide the details. Although we don’t know yet what changes, if any, will happen to the KN concurrency and state model to accomodate coroutines, unless Jetbrains radically changes their plan and abandons everything, you’ll definitely need to understand this stuff.